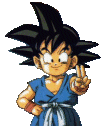
Vy Thanh Định- Web Master

- Giới tính :
Bài gửi : 228
Tổng Điểm : 544
Điểm Thưởng : 16
Sinh Nhật : 19/05/1990 Bị Dụ Dỗ : 11/09/2009
Tuổi : 34
by Vy Thanh Định 29/11/2009, 22:39
- Code:
#include <iostream.h>
#include <stdlib.h>
struct NODE
{
int data;
NODE *next;
};
typedef struct NODE *LIST;
void InsertFirst(LIST *l, int x)
{
LIST p = (LIST)malloc(sizeof(struct NODE));
p->data = x;
p->next = *l;
*l = p;
}
void InsertLast(LIST *l, int x)
{
LIST p = (LIST)malloc(sizeof(struct NODE));
p->data = x;
p->next = NULL;
if(!*l) *l = p;
else
{
LIST q;
for(q = *l; q->next; q=q->next)
{}
q->next = p;
}
}
/*
void InsertLastDeQuy(LIST *l, int x)
//chu y: cach nay chi dung voi danh sach co so luong node it
{
if(!*l)//hoac (*l == NULL)
{
*l = (LIST)malloc(sizeof(struct NODE));
*l->data = x;
*l->next = NULL;
return;
}
InsertLastDeQuy(&(*l)->next, x);
}
void AfterNodeInsert(LIST l, LIST q, int x)
{
if(l && q)
{
LIST p = (LIST)malloc(sizeof(struct NODE));
p->data = x;
p->next = q->next;
q->next = p;
}
}
*/
void AfterInsert(LIST l, int d, int x)
{
if(!l) return;
if(l->data == d)
{
LIST p = (LIST)malloc(sizeof(struct NODE));
p->data = x;
p->next = l->next;
l->next = p;
return;
}
AfterInsert(l->next, d, x);
}
void Outlist(LIST l,char *s)
{
cout<<s<<" : ";
for(; l; l = l->next)
cout<<l->data<<" ";
cout<<"\n";
}
int main()
{
LIST l = NULL;
int x, n;
cout<<"Nhap so phan tu: ";
cin>>n;
for(int i = 0; i < n; i++)
{
cout<<"x["<<i<<"]: ";
cin>>x;
InsertFirst(&l,x);
}
Outlist(l,"List goc ");
int cuoi;
cout<<"Nhap gia tri chen vao cuoi: ";
cin>>cuoi;
InsertLast(&l, cuoi);
Outlist(l,"Chen 5 cuoi ");
int tim, chen;
cout<<"Gia tri muon chen: "; cin>>chen;
cout<<"Sau gia tri: "; cin>>tim;
AfterInsert(l, tim, chen);
Outlist(l,"List sau khi chen");
return 0;
}